Hi,
Here are two ideas. The first is simpler. The second is more flexible.
First idea:
This idea doesn't require any custom scripts or custom fields in your dialogue database.
Set each quest's Name to the same as the actor's Name, following
these rules (mainly, replace blank spaces with underscores). Set the quest's Display Name to the actual quest name that appears in quest UIs. Example with NPC named "Innkeeper" and quest "Kill Rats":
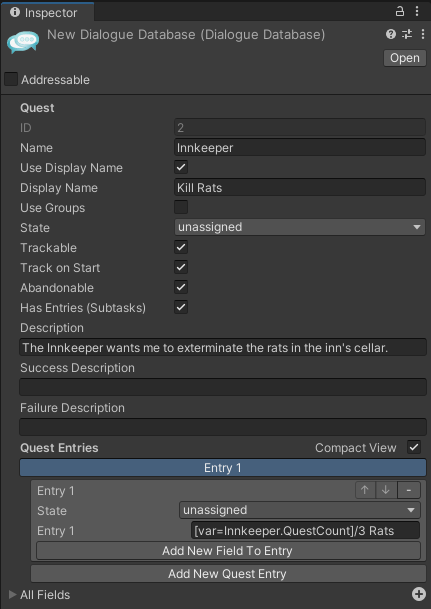
- quests1.png (37.73 KiB) Viewed 320 times
Let's say you use a generic conversation in which you assign the Innkeeper as the conversation's conversant. When the conversation starts, the Dialogue System will set the variable Variable["ConversantIndex"] to the Name of the conversant (e.g., "Innkeeper"). Then you can use this Lua code in a dialogue entry's Script field:
Code: Select all
SetQuestState(Variable["Conversant"], active);
ShowAlert("New Quest: " .. Quest[Variable["ConversantIndex"]].Display_Name)
For the Innkeeper, the first line will translate to SetQuestState("Innkeeper", active). (The Dialogue System defines constants unassigned, active, success, etc, so you can use those constants or the literal string values "unassigned", "active", "success", etc.)
The second line will translate to ShowAlert("New Quest: " .. Quest["Innkeeper"].Display_Name), which will further boil down to ShowAlert("New Quest: Kill Rats").
---
Second idea:
Every "asset" type (actor, quest, conversation, etc.) in a dialogue database can have custom fields. For example, you could add a "Quest Giver" field to your quests (or quest
template):
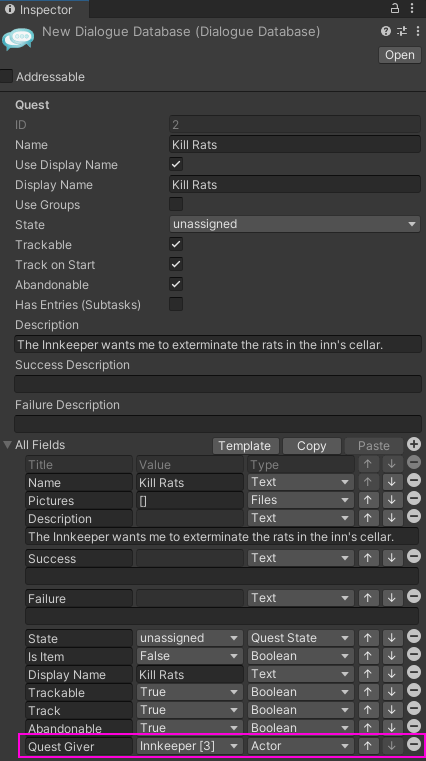
- quests2.png (53.41 KiB) Viewed 320 times
Alternatively, you could add a field to the actor:
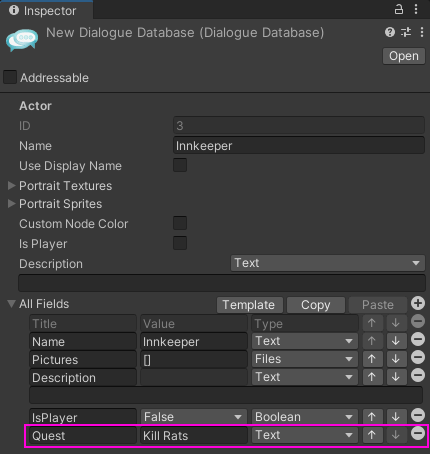
- quests3.png (30.84 KiB) Viewed 320 times
Then you can look up an actor by its quest, or a quest by its actor:
Code: Select all
SetQuestState(Actor[Variable["ConversantIndex"]].Quest, active);
questDisplayName = Quest[Actor[Variable["ConversantIndex"]].Quest].Display_Name;
ShowAlert("New Quest: " .. questDisplayName)
You can get even more sophisticated by adding fields to your quest template that you handle in a standard way. In the recently released Gestalt: Steam & Cinder, we defined quest fields that were similar to this:
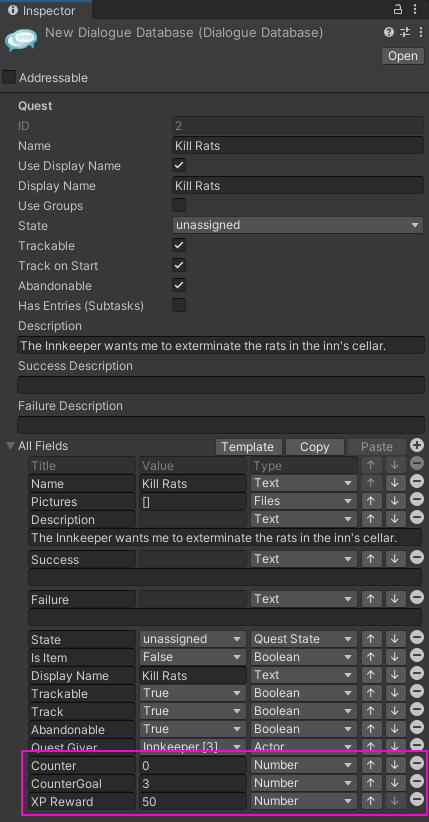
- quests4.png (57.12 KiB) Viewed 320 times
Then we could programmatically increment the quest's "Counter" field (e.g., using DialogueLua.SetQuestField()), check if it has reached the "CounterGoal" value, and then set the state to success and grant the "XP Reward". There are more fields than this in Gestalt's quest template, such as a custom field type for item rewards, but this should be enough to give you an idea.
Other studios have also added custom quest fields that contain Lua code -- for example, a custom field containing Lua code to run when the player accepts the quest. This code can spawn GameObjects or otherwise set up the beginning of the quest. Or, for another example, a custom field containing Lua code to run when the player completes the quest. That code could
run C# methods that give rewards to the player.