Page 1 of 1
visible variables in the UI
Posted: Thu Jan 04, 2024 11:33 am
by tohaMem
Hello!
I'm in the process of creating a level up system. It's based on the number of points received in the dialogue.
How can I display this variables in the UI? Something like this, but only in numbers, yes
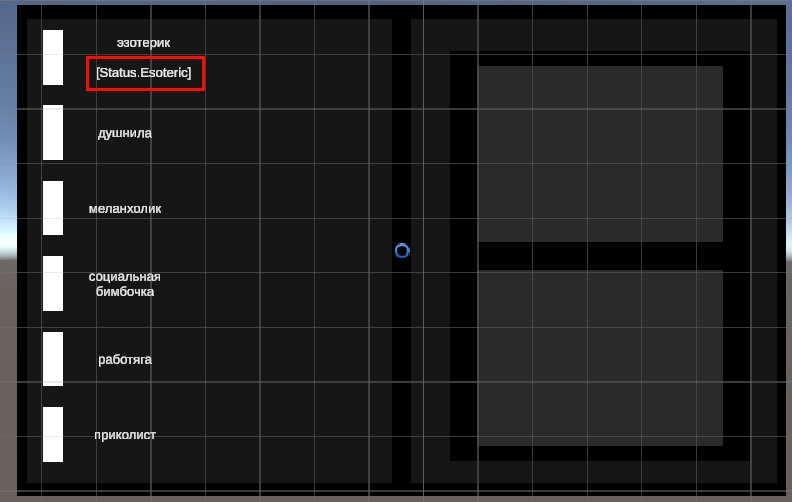
Re: visible variables in the UI
Posted: Thu Jan 04, 2024 2:13 pm
by Tony Li
Hi,
If you want to show a variable's value in dialogue text, use the [var=
variable]
markup tag. Example:
- Dialogue Text: "Your esoteric status is [var=Status.Esoteric]."
To replace [var=
variable] tags in other strings, use
FormattedText.ParseCode(). Example:
Code: Select all
MyTextMeshProUGUI.text = FormattedText.ParseCode("[var=Status.Esoteric]");
However, if you only need the numeric value, it's easiest in C# to use
DialogueLua.GetVariable(). Example:
Code: Select all
MyTextMeshProUGUI.text = DialogueLua.GetVariable("Status.Esoteric").asString;
Re: visible variables in the UI
Posted: Thu Jan 04, 2024 5:31 pm
by tohaMem
oh, thank you! All worked!
And another question! How can I extract variables from DS and add/subtract them in C#?
Re: visible variables in the UI
Posted: Thu Jan 04, 2024 8:24 pm
by Tony Li
Hi,
Use DialogueLua.GetVariable() and DialogueLua.SetVariable(). Example:
Code: Select all
// Add +1 to Esoteric:
DialogueLua.SetVariable(DialogueLua.GetVariable("Status.Esoteric").asInt + 1);
Alternatively, you can make a property:
Code: Select all
public int Esoteric
{
get { return DialogueLua.GetVariable("Status.Esoteric").asInt; }
set { DialogueLua.SetVariable("Status.Esoteric", value); }
}