Setting the state of a Quest
-
- Posts: 17
- Joined: Sat Jan 23, 2016 1:35 pm
Setting the state of a Quest
I'm just trying to figure out how to set the state of a Quest to 'Success', after a variable reaches a certain number. In this case, I have a variable called 'Kills'. When I get 1 kill, I want the state of the Quest to be set to 'Success'. The variable is tracked successfully, because when I check the Quest Log, It says '1 of 1 kills', but the Quest is still set as active. What would I need to use in order to set the state? There's no conversation involved with this Quest. I know how to set states through conversation, but I'm just not sure how to set the state in this case.
Re: Setting the state of a Quest
Hi,
If you've added an Increment On Destroy component to the kill target(s), you could also add a Quest Trigger configured like this:
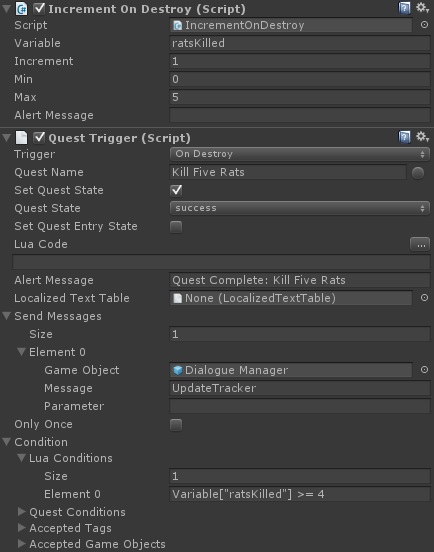
If you want to set additional Lua variables, you can use the Lua Code section -- for example to update relationship functions, call your own registered functions (e.g., give experience points), etc.
Alternatively, you can replace Increment On Destroy and Quest Trigger with the "universal" trigger component, Dialogue System Trigger.
If you've added an Increment On Destroy component to the kill target(s), you could also add a Quest Trigger configured like this:
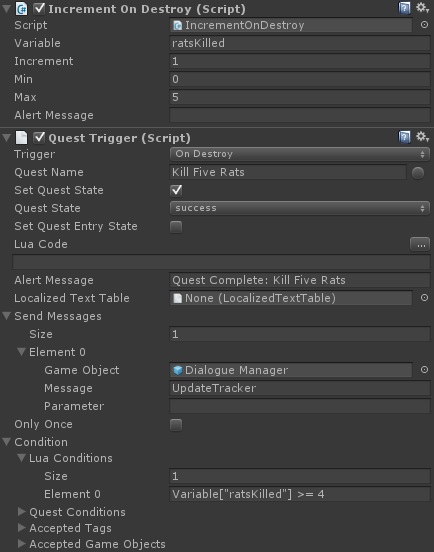
- Trigger dropdown: On Destroy.
- Quest State: success.
- Alert Message: (Optional) Show an alert message.
- Send Messages: Send "UpdateTracker" to the Dialogue Manager. [Note: In v1.5.9 coming out tomorrow you can skip this; it will be automatic.]
- Lua Conditions: Check if there are already enough kills that this kill will put it over the requirement.
If you want to set additional Lua variables, you can use the Lua Code section -- for example to update relationship functions, call your own registered functions (e.g., give experience points), etc.
Alternatively, you can replace Increment On Destroy and Quest Trigger with the "universal" trigger component, Dialogue System Trigger.
-
- Posts: 17
- Joined: Sat Jan 23, 2016 1:35 pm
Re: Setting the state of a Quest
Instead of Increment On Destroy, I created a modified version of it, as the enemies in my game won't be destroyed, when killed.
This is just a snippet of the code, where the value is incremented when the enemy is killed:
It's the same code as the Increment On Destroy class except, it doesn't check for when something is destroyed. It just checks for when the health of the enemy is 0 and then increments the value and displays the alert. The tracking of the values works, but I can't figure out how to get the Quest State to be set to 'Success'. What would I need to do in order to set the state of the quest this way, instead of using Increment On Destroy? Would the Dialogue System Trigger be used for this also, or would something else need to be done in this case?
This is just a snippet of the code, where the value is incremented when the enemy is killed:
Code: Select all
void Update()
{
if(enemAI.health <= 0)
{
if(!killed)
{
int oldValue = DialogueLua.GetVariable(ActualVariableName).AsInt;
int newValue = Mathf.Clamp(oldValue + increment, min, max);
DialogueLua.SetVariable(ActualVariableName, newValue);
DialogueManager.SendUpdateTracker();
if (!(string.IsNullOrEmpty(alertMessage) || DialogueManager.Instance == null))
{
DialogueManager.ShowAlert(alertMessage);
}
killed = true;
}
}
}
It's the same code as the Increment On Destroy class except, it doesn't check for when something is destroyed. It just checks for when the health of the enemy is 0 and then increments the value and displays the alert. The tracking of the values works, but I can't figure out how to get the Quest State to be set to 'Success'. What would I need to do in order to set the state of the quest this way, instead of using Increment On Destroy? Would the Dialogue System Trigger be used for this also, or would something else need to be done in this case?
Re: Setting the state of a Quest
Since you have your own script, it's even easier because you can use QuestLog directly. Before the "DialogueManager.SendUpdateTracker()" line, add something like this:
(QuestLog.CompleteQuest("foo") is just a shortcut for QuestLog.SetQuestState("foo", QuestState.Success).)
Code: Select all
if (newValue >= requiredAmount) QuestLog.CompleteQuest("My Quest Title");