Hi,
Thanks for checking out the Dialogue System!
I'll outline some different approaches. If one of them sounds interesting to you, I can describe it in more detail.
1. Write a
custom sequencer command that uses a string. For example, the dialogue entry's Sequence field might look like:

- animancer1.png (2.4 KiB) Viewed 1947 times
The sequencer command would roughly look like:
Code: Select all
public class SequencerCommandPlayAnimancer : SequencerCommand
{
void Awake()
{
var enumStringName = GetParameter(0); // First parameter is text name of enum.
var playerPuppeteerAnimationClip = GetSubject(1, speaker); // Second param is object with PlayerPuppeteeerAnimationClip (defaults to node's speaker).
foreach (var enumValue in Enum.GetValues(typeof(AnimEnum)))
{
if (enumStringName == enumValue.ToString())
{
playerPuppeteerAnimationClip.Play(enumValue);
break;
}
}
Stop();
}
}
This is the quickest to implement, but the downside is that it depends on string names instead of the actual enum values.
2. Or you can add a
custom field to the dialogue entry template. Custom fields can have their owner drawers, which can use a dropdown:
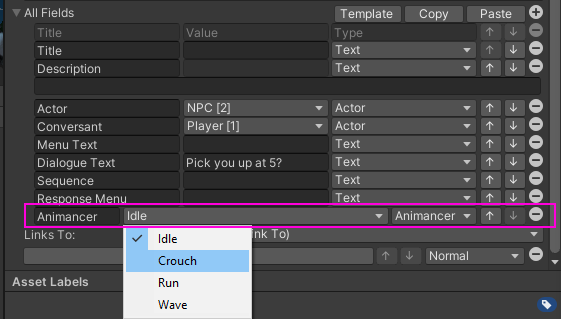
- animancer2.png (28.02 KiB) Viewed 1947 times
Then you can add an OnConversationLine that checks this field and, if set, plays the corresponding animation through Animancer.
3. Or you can change your PlayerPuppeteerAnimationClip script to use ScriptableObjects as extensible enums instead of using a C# enum. For example, your ScriptableObject might look like:
Code: Select all
[CreateAssetMenu] public class AnimancerClip : ScriptableObject
{
public AnimationClip animationClip;
}
with ScriptableObject assets in your project named Crouch, Idle, Walk, etc.
The relevant code in your PlayerPuppeteerAnimationClip script might be changed to:
Code: Select all
public void Play(AnimancerClip animancerClip)
{
_Animancer.Play(animancerClip.animcationClip);
}
Then you can use dialogue entry nodes' OnExecute() UnityEvents, and assign the desired ScriptableObject asset.