Hidden Value system and a thanks
Hidden Value system and a thanks
First, I wanted to thank you guys. You helped me get started for a proposal to show my class I could make use of this dialogue system better than traditional c# scripting (unfortunately, I still had to build it to prove my point).
Next, I've set up a hidden value system that scores each response. For example, your A, B, C, D responses to the NPC each carry their own respective weighted value that I want to be hidden from the user. Is there a way to set a value for each response and maybe add a listener to the responses once they are clicked that would then compare against a preset winning score at the end of the player and NPC's conversation.
If that didn't make sense I will check back in to clear things up!.
Thanks again!
Next, I've set up a hidden value system that scores each response. For example, your A, B, C, D responses to the NPC each carry their own respective weighted value that I want to be hidden from the user. Is there a way to set a value for each response and maybe add a listener to the responses once they are clicked that would then compare against a preset winning score at the end of the player and NPC's conversation.
If that didn't make sense I will check back in to clear things up!.
Thanks again!
Re: Hidden Value system and a thanks
Hi,
On the Dialogue Editor's Variables tab, define a variable in your dialogue database, for example named "Score".
In each response, use the Script field to increment the variable by the desired amount. You can use the point-and-click Lua wizard (as shown below) or enter the Lua code by hand.
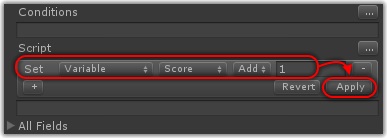
Then check the value at some point. You could check it later in the conversation using the Conditions field, or use a Condition Observer component to check the value on a regular basis, or add a script that uses a Lua observer to watch the value.
On the Dialogue Editor's Variables tab, define a variable in your dialogue database, for example named "Score".
In each response, use the Script field to increment the variable by the desired amount. You can use the point-and-click Lua wizard (as shown below) or enter the Lua code by hand.
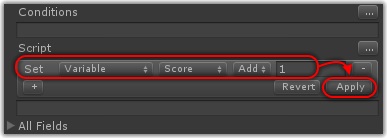
Then check the value at some point. You could check it later in the conversation using the Conditions field, or use a Condition Observer component to check the value on a regular basis, or add a script that uses a Lua observer to watch the value.
Re: Hidden Value system and a thanks
Would I need to script something to have that score trigger a pop-out if the right conditions are met?
Re: Hidden Value system and a thanks
You only need to script if that's your preference. Pretty much anything you can do in the Dialogue System with scripting you can also do with a visual scripting tool such as PlayMaker or with some simpler techniques I'll describe below:
I'm not sure what you mean by pop-out, but let's say it's a Unity UI panel that stays offscreen until the player wins. You could add an Animator component to the panel with a Show state. The Show state would animate the panel's position to move it onscreen. In this case, you can use the AnimatorPlay() sequencer command to play the Show state.
For example, say the panel is named "Win Panel". It has an Animator with an animator controller that has two states: Nothing (the default) and Show. When the right conditions are met, play this sequence:
Since you only need to check the score after the player has chosen a response, Condition Observer is kind of inefficient, since it runs continuously.
The most efficient way would be to check the score after each answer. In your conversation, create a single win node. Set its Conditions field to check if the right conditions are met. Set the Sequence field to the sequence above.
The only catch is that the Dialogue System always evaluates one level ahead in the conversation. So you'll have to add an intermediate node to delay the evaluation until after the player has selected a response, like this:
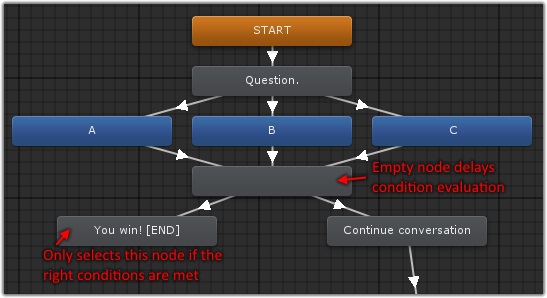
I'm not sure what you mean by pop-out, but let's say it's a Unity UI panel that stays offscreen until the player wins. You could add an Animator component to the panel with a Show state. The Show state would animate the panel's position to move it onscreen. In this case, you can use the AnimatorPlay() sequencer command to play the Show state.
For example, say the panel is named "Win Panel". It has an Animator with an animator controller that has two states: Nothing (the default) and Show. When the right conditions are met, play this sequence:
Code: Select all
AnimatorPlay(Show, Win Panel)
The most efficient way would be to check the score after each answer. In your conversation, create a single win node. Set its Conditions field to check if the right conditions are met. Set the Sequence field to the sequence above.
The only catch is that the Dialogue System always evaluates one level ahead in the conversation. So you'll have to add an intermediate node to delay the evaluation until after the player has selected a response, like this:
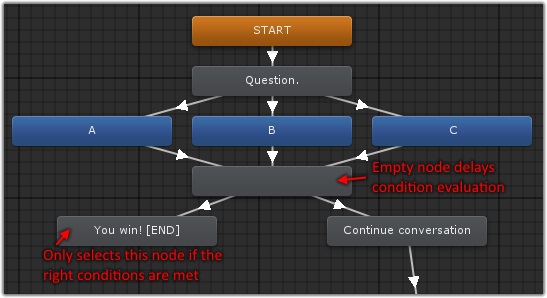
Re: Hidden Value system and a thanks
Thanks! this should help a TON. Could you possibly point me towards the documentation for the variables tab in the documentation. I'm not getting a script component like your screenshot or is that in the inspector window I could be looking over it(I checked both)

Re: Hidden Value system and a thanks
The Conditions and Script fields are on each dialogue entry node in a conversation, which you edit on the Conversations tab.
Take for example the conversation pictured above with the orange START node, gray subtitle nodes, and blue player response nodes:
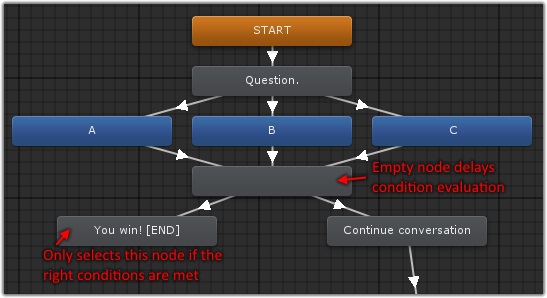
Say answer "A" is correct and awards one point. If you click on the "A" node, the Inspector will show the node's details, including its Script field, which you could use to increment the "Score" variable by 1:
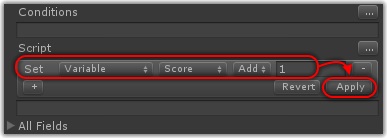
Similarly, if you click on the "You win!" node, you can set the Conditions field to something like:
So the Dialogue System will only choose "You win!" if the player's Score is over 9.
I just thought of one last thing. I didn't mention this earlier, but the dialogue tree image assumes that "You win!" comes before "Continue conversation..." in the list of links. You can order them in the previous node's Inspector using the up and down arrow buttons next to each link. You can also set the Priority of each link to force the Dialogue System to evaluate certain nodes before others regardless of order. To do this, click on the link arrow; then select the priority from the dropdown menu in the Inspector.
Take for example the conversation pictured above with the orange START node, gray subtitle nodes, and blue player response nodes:
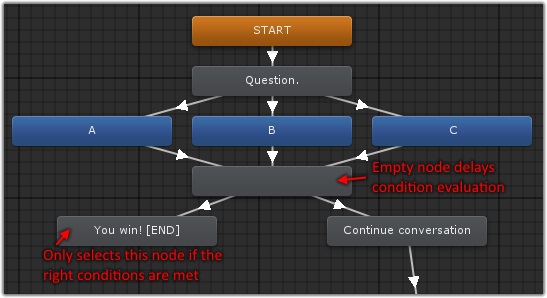
Say answer "A" is correct and awards one point. If you click on the "A" node, the Inspector will show the node's details, including its Script field, which you could use to increment the "Score" variable by 1:
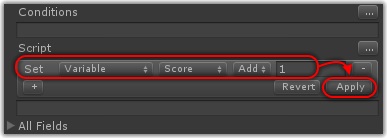
Similarly, if you click on the "You win!" node, you can set the Conditions field to something like:
Code: Select all
Variable["Score"] > 9
I just thought of one last thing. I didn't mention this earlier, but the dialogue tree image assumes that "You win!" comes before "Continue conversation..." in the list of links. You can order them in the previous node's Inspector using the up and down arrow buttons next to each link. You can also set the Priority of each link to force the Dialogue System to evaluate certain nodes before others regardless of order. To do this, click on the link arrow; then select the priority from the dropdown menu in the Inspector.
Re: Hidden Value system and a thanks
Thank You!!!! I really appreciate it!
Re: Hidden Value system and a thanks
Happy to help! Don't hesitate to ask for more clarification if you get stuck on anything. The Conditions and Script fields can be complicated, but they really give you a lot of power.
Re: Hidden Value system and a thanks
Everything is working and integrated with my existing project!!!
I realized I didn't explain what I meant by pop-outs very well.
For instance, lets say your using a UI button in unity and you use that button's on-click to set an inactive image on screen to active (using SetActive to true). In case that didn't make sense hopefully this clears me up.
.
Is there a better way using your system? maybe add a continue button to the gui I made for those particular instances?
I realized I didn't explain what I meant by pop-outs very well.
For instance, lets say your using a UI button in unity and you use that button's on-click to set an inactive image on screen to active (using SetActive to true). In case that didn't make sense hopefully this clears me up.

Is there a better way using your system? maybe add a continue button to the gui I made for those particular instances?
Re: Hidden Value system and a thanks
What about using the SetActive() sequencer command? Something like:
You can use sequencer commands in dialogue entries' Sequence fields, in Sequence Triggers (and other triggers such as the general purpose Dialogue System Trigger), and Condition Observers.
Code: Select all
SetActive(PopOut)