Page 1 of 3
Access variable from c#
Posted: Tue Jul 26, 2022 3:31 pm
by hrohibil
Hello
If I set a variable in Dialogue manager, how do get access to it from a C# script?
Thanks
Re: Access variable from c#
Posted: Tue Jul 26, 2022 4:28 pm
by Tony Li
Hi,
Use
DialogueLua.GetVariable(). Example:
Code: Select all
using PixelCrushers.DialogueSystem; // (Put at top of script)
...
bool value = DialogueLua.GetVariable("My Variable").asBool;
Re: Access variable from c#
Posted: Wed Jul 27, 2022 6:35 am
by hrohibil
Hi Tony
I tried like this and attached it to an empty GameObject.
But i get this console error: shown image below
Code: Select all
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using PixelCrushers.DialogueSystem;
public class getDialogueVariable : MonoBehaviour
{
bool value = DialogueLua.GetVariable("AnimatorPlay").asBool;
public GameObject mobileUI;
void Update()
{
if (value == true)
{
mobileUI.SetActive(false);
}
else if (value == false)
{
mobileUI.SetActive(true);
}
}
}
Re: Access variable from c#
Posted: Wed Jul 27, 2022 8:19 am
by Tony Li
Move this line:
Code: Select all
bool value = DialogueLua.GetVariable("AnimatorPlay").asBool;
into the Update method:
Code: Select all
void Update()
{
bool value = DialogueLua.GetVariable("AnimatorPlay").asBool;
However, you shouldn't need to check the variable every frame. It's not efficient and may severely impact your framerate on mobile devices. It looks like this might be related to your other question about disabling GameObjects. If so, use that instead of this script.
Re: Access variable from c#
Posted: Wed Jul 27, 2022 8:55 am
by hrohibil
Thank you.
I get it would be a performance hit when in update. How would you check then in a C# scripts to see if the bool is true/false?
Re: Access variable from c#
Posted: Wed Jul 27, 2022 9:01 am
by Tony Li
You can do the check; just don't do it every single frame in Update if you can find another way.
Re: Access variable from c#
Posted: Wed Jul 27, 2022 6:13 pm
by hrohibil
Ok thanks Tony.
I have a dialogue where NPC ask me if i have a key. I have set a variable gotKey for this.
But how do i actually when i get an item say the key set the gotKey variable to true?
Re: Access variable from c#
Posted: Wed Jul 27, 2022 8:55 pm
by Tony Li
Since you're using Invector, don't use a gotKey variable. Use the special
Invector Lua function vGetItemCount(). For example, say your Invector item has ID 15. Then your conversation might look something like:
- NPC Dialogue Text: "Do you have the key?"
- Player Dialogue Text: "Yes."
Conditions: vGetItemCount(15) > 0
- Player Dialogue Text: "No."
The response "Yes" will only be available if the player has a key.
Re: Access variable from c#
Posted: Wed Jul 27, 2022 9:07 pm
by hrohibil
Thanks Tony.
How awesome the integration with Invector is so nicely crafted..
BTW:
With the lua method can I still in a conversation check for a condition to see the key is obtain?
Re: Access variable from c#
Posted: Wed Jul 27, 2022 9:24 pm
by Tony Li
Yes:
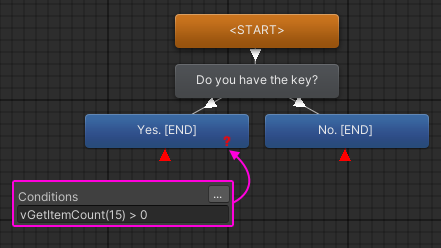
- invectorConditions.png (14.05 KiB) Viewed 2397 times