Page 1 of 2
Add custom audio clip array to actor entry in database (like portraits)
Posted: Fri Feb 11, 2022 11:35 am
by OddMan2
Hello,
i need to assign an array of sound effects to each actor in the database, in the same fashion as portraits.
my use case is the following;
i have a retro- style system that plays a random audio clip every time the typewriter script prints a character in the subtitle ui.
The audio clip is choosen from an array. I would like to change the array according to the actor that is speaking, so they have different "voices".
What is the best approach to achieve this? i read the documentation on custom fields but they are just for text from what i understand?
Thank you for your help!
Re: Add custom audio clip array to actor entry in database (like portraits)
Posted: Fri Feb 11, 2022 1:54 pm
by Tony Li
Hi,
Make a subclass of
DialogueActor that has your audio clip array:
Code: Select all
public class AudibleDialogueActor : DialogueActor
{
public AudioClip[] typewriterAudioClip;
public AudioClip[] alternateTypewriterAudioClips;
}
Add this to your characters' GameObjects. If a character doesn't have a GameObject, you can create an empty GameObject for it.
Then add a script with an
OnConversationLine method to the Dialogue Manager GameObject:
Code: Select all
public class SetTypewriterAudioForActor : MonoBehaviour
{
void OnConversationLine(Subtitle subtitle)
{
DialogueActor dialogueActor;
var panel = DialogueManager.standardDialogueUI.conversationUIElements.standardSubtitleControls.GetPanel(subtitle, out dialogueActor);
var audibleActor = dialogueActor as AudibleAudioActor;
var typewriter = panel.GetTypewriter();
typewriter.audioClip = audibleActor.typewriterAudioClip;
typewriter.alternateAudioClips = audibleActor.alternateTypewriterAudioClips;
}
}
Note: I just typed that into the reply, so there might be typos. For clarity, I also omitted using statements at the top and error checking for null when getting panels, actors, etc.
Re: Add custom audio clip array to actor entry in database (like portraits)
Posted: Fri Feb 11, 2022 5:19 pm
by OddMan2
Hi Tony,
Thank you so much for your answer!
i managed to make it work, but for some reason the serialized array in the sublcass is not visible in the inspector, and i can't seem to figure out why. Any ideas?
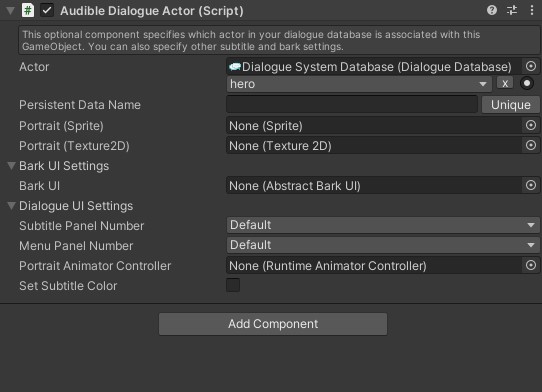
Re: Add custom audio clip array to actor entry in database (like portraits)
Posted: Fri Feb 11, 2022 5:32 pm
by Tony Li
DialogueActor has a custom inspector script.
Create a folder named Editor, and put this script in it:
AudibleDialogueActorEditor.cs
Code: Select all
using UnityEditor;
namespace PixelCrushers.DialogueSystem
{
[CustomEditor(typeof(AudibleDialogueActor), true)]
[CanEditMultipleObjects]
public class AudibleDialogueActorEditor : DialogueActorEditor
{
public override void OnInspectorGUI()
{
base.OnInspectorGUI();
serializedObject.Update();
EditorGUILayout.PropertyField(serializedObject.FindProperty(nameof(AudibleDialogueActor.typewriterAudioClip)), true);
EditorGUILayout.PropertyField(serializedObject.FindProperty(nameof(AudibleDialogueActor.alternateTypewriterAudioClips)), true);
serializedObject.ApplyModifiedProperties();
}
}
}
Re: Add custom audio clip array to actor entry in database (like portraits)
Posted: Fri Feb 11, 2022 5:51 pm
by OddMan2
Oh i missed that.
Now it works,
thank you for your great (and very quick) support!
Re: Add custom audio clip array to actor entry in database (like portraits)
Posted: Fri Feb 11, 2022 9:27 pm
by Tony Li
Glad to help!
Re: Add custom audio clip array to actor entry in database (like portraits)
Posted: Wed Jul 27, 2022 4:38 pm
by moetan14
Reviving this thread since I wanted to implement this solution, as it seems to be the most comfy for situations like this.
I encountered this issue:
https://a.uguu.se/WHXyMAdU.mp4
Can't assign the files to the array in any way. It won't let me. The individual clip behaves like this as well; no matter how much times I try to drag and drop the file into the slot it just won't accept it. The little circle doesn't work either.
Anything I can do to solve this?
Re: Add custom audio clip array to actor entry in database (like portraits)
Posted: Wed Jul 27, 2022 5:14 pm
by Tony Li
Hi,
That's strange. I've never seen that before. Does it still happen if you close and re-open Unity? What Unity version are you using?
As a test, can you try adding a typewriter effect to a new, empty GameObject in the scene, and then try assigning audio clips to it? Maybe this will point out some difference.
Re: Add custom audio clip array to actor entry in database (like portraits)
Posted: Wed Jul 27, 2022 5:45 pm
by moetan14
I'm using 2021.3.0f1 - still happens when I restart Unity.
Made a new object and gave it Unity UI Typewriter Effect, and was able to assign files just fine. It's only the Audible Dialogue Actor that's giving me static. Every other component allows me to drag and drop objects as normal.
I did make some changes that I don't know if they have anything to do.
Code: Select all
var audibleActor = dialogueActor as AudibleAudioActor;
became
Code: Select all
var audibleActor = dialogueActor as AudibleDialogueActor;
(gives me an error otherwise)
Code: Select all
public AudioClip[] typewriterAudioClip;
public AudioClip[] alternateTypewriterAudioClips;
became
Code: Select all
public AudioClip typewriterAudioClip;
public AudioClip[] alternateTypewriterAudioClips;
(throws an error otherwise as well, saying it can't convert audioclip[] to audioclip)
Re: Add custom audio clip array to actor entry in database (like portraits)
Posted: Wed Jul 27, 2022 8:52 pm
by Tony Li
I just noticed there was a typo in the script above. The second "serializedObject.Update();" should be:
Code: Select all
serializedObject.ApplyModifiedProperties();
I just fixed in in the script above, too.