Tip: Managing Spawnpoints
Posted: Wed Dec 13, 2017 2:00 pm
Someone asked some good questions about spawnpoints in an email.
In the LoadLevel() sequencer command, you can specify where the player will spawn in the new level. For example:
will load New Level and place the player at the position of the GameObject named "Spawnpoint From Old Level".
The LoadLevel() command sets the actor named "Player"'s Spawnpoint field. In Lua, that field is:
(Spawnpoints can apply to any object. If the object has a Persistent Position Data component, it will check for an actor field named "Spawnpoint". Further down in this tip, I'll explain how to set spawnpoints for other characters, too.)
A few screenshots will help illustrate how this works. In the example, there are two scenes: MAINSCENE and ANOTHERSCENE_UMA.
The screenshot below is in the first scene (MAINSCENE). The "Portal to ANOTHERSCENE" GameObject has a Sequence Trigger with a LoadLevel() command to load ANOTHERSCENE and position the player at "Spawnpoint from MAINSCENE":
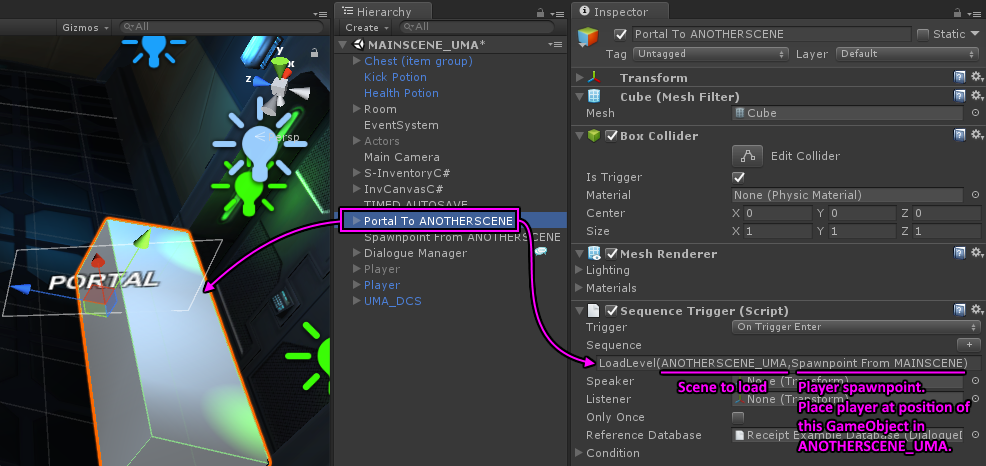
The screenshot below is in ANOTHERSCENE_UMA. It shows where "Spawnpoint from MAINSCENE" is. This is where the player will appear:
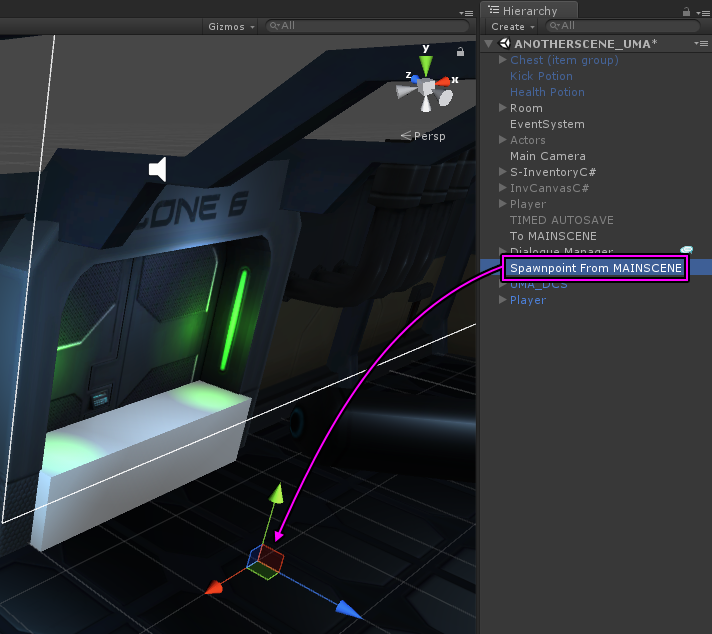
You can manually set spawnpoints for other objects before loading the level. For example:
To do this, you may want to use a Dialogue System Trigger instead of a Sequence Trigger. This will allow you to run Lua code to set their Spawnpoint fields before loading the next scene:
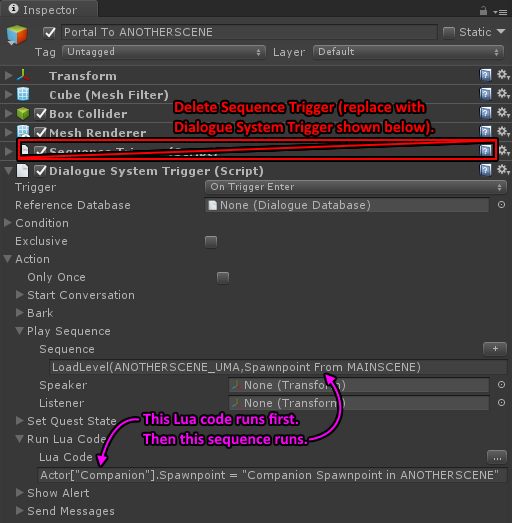
In the upcoming version 1.7.7, the order in the Action section (Run Lua Code, Play Sequence, etc.) will be changed to accurately reflect the order in which they're executed.
The Persistent Position Data component clears the Spawnpoint field after using it. However, if for some reason a spawnpoint isn't used, it won't be cleared. Before you save a game, you can clear the spawnpoints manually:
Or automatically using a script like this:
ClearSpawnpointsInSavedGames.cs
In the LoadLevel() sequencer command, you can specify where the player will spawn in the new level. For example:
Code: Select all
LoadLevel(New Level, Spawnpoint From Old Level)
The LoadLevel() command sets the actor named "Player"'s Spawnpoint field. In Lua, that field is:
Code: Select all
Actor["Player"].Spawnpoint = "Spawnpoint from Old Level"
A few screenshots will help illustrate how this works. In the example, there are two scenes: MAINSCENE and ANOTHERSCENE_UMA.
The screenshot below is in the first scene (MAINSCENE). The "Portal to ANOTHERSCENE" GameObject has a Sequence Trigger with a LoadLevel() command to load ANOTHERSCENE and position the player at "Spawnpoint from MAINSCENE":
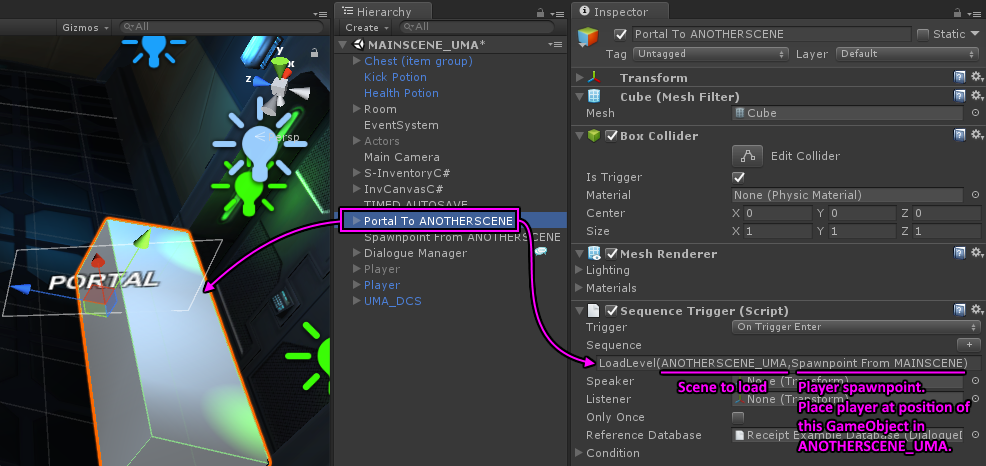
The screenshot below is in ANOTHERSCENE_UMA. It shows where "Spawnpoint from MAINSCENE" is. This is where the player will appear:
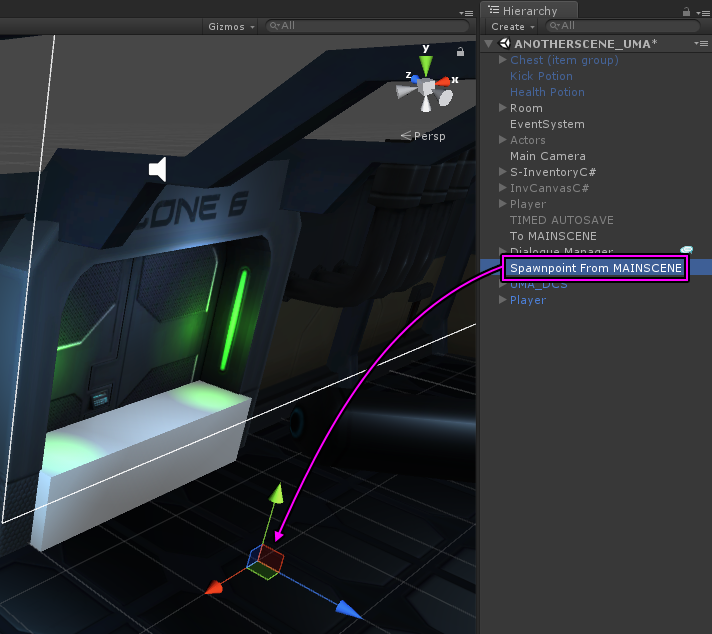
You can manually set spawnpoints for other objects before loading the level. For example:
Code: Select all
Actor["Second Player"].Spawnpoint = "Second Spawnpoint In New Level";
Actor["Companion"].Spawnpoint = "Companion Spawnpoint In New Level";
etc.
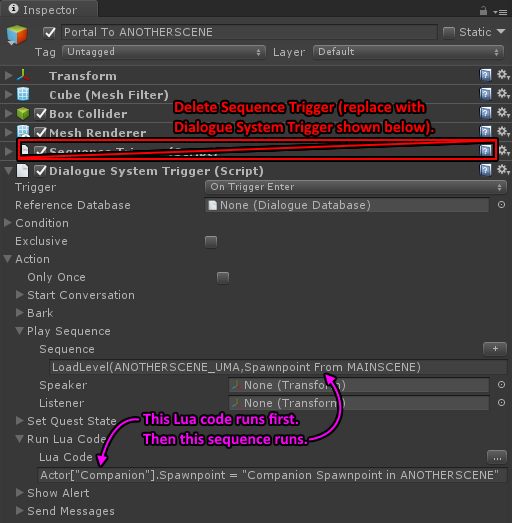
In the upcoming version 1.7.7, the order in the Action section (Run Lua Code, Play Sequence, etc.) will be changed to accurately reflect the order in which they're executed.
The Persistent Position Data component clears the Spawnpoint field after using it. However, if for some reason a spawnpoint isn't used, it won't be cleared. Before you save a game, you can clear the spawnpoints manually:
Code: Select all
Actor["Player"].Spawnpoint = "";
Actor["Second Player"].Spawnpoint = "";
Actor["Companion"].Spawnpoint = "";
ClearSpawnpointsInSavedGames.cs
Code: Select all
using UnityEngine;
using PixelCrushers.DialogueSystem;
public class ClearSpawnpointsInSavedGames : MonoBehaviour
{
void Start()
{
PersistentDataManager.GetCustomSaveData = GetClearSpawnpointsCode;
}
string GetClearSpawnpointsCode()
{
string s = string.Empty;
foreach (var actor in DialogueManager.MasterDatabase.actors)
{
var actorIndex = DialogueLua.StringToTableIndex(actor.Name);
s += "Actor['" + actorIndex + "'].Spawnpoint = ''; ";
}
return s;
}
}