Hi.
I am new with the use of this system, my problem is that I want to show variables that are in my scripts in a conversation.
For example, when I open a chest, I want to show "The chest contains axe". But "axe" is a variable that corresponds to the name of the item in the chest, which I extract from a database.
My question is: How could I access a variable that is in another script to be shown in the conversation?
Thank you so much.
PS: Sorry for my level of English.
Scripts variables in Conversations
Re: Scripts variables in Conversations
Hi,
Option 1:
In Dialogue Text and Menu Text fields, you can use markup tags.
One of these markup tags is the [var=variableName] tag. At runtime, the Dialogue System will replace the tag with the value of a variable in your dialogue database.
For example, say you define a variable named chestContents with a value of "Axe":
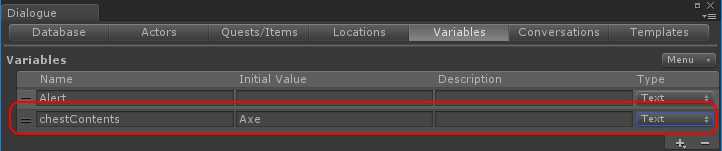
Then you can set Dialogue Text to:

At runtime, the conversation will show "The chest contains Axe."
You can set the dialogue database variable in your own C# or UnityScript script with this code:
Option 2:
At runtime, the Dialogue System keeps its data in Lua. In most cases, you don't have to think about this. But sometimes it's helpful to use Lua. For example, you can use the [lua(...)] tag in Dialogue Text:

This is a very powerful feature because you can register your own C# code with Lua. (There is a starter template script in Assets / Dialogue System / Scripts / Templates.)
For example, you can register a function named GetChestContents() that returns the name of the object inside the chest:
Then you can use that function in your Dialogue Text:

Option 1:
In Dialogue Text and Menu Text fields, you can use markup tags.
One of these markup tags is the [var=variableName] tag. At runtime, the Dialogue System will replace the tag with the value of a variable in your dialogue database.
For example, say you define a variable named chestContents with a value of "Axe":
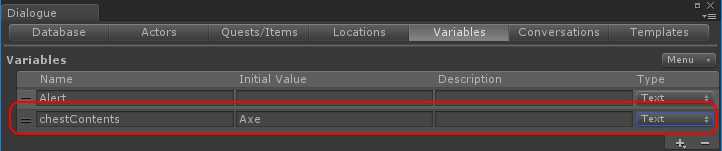
Then you can set Dialogue Text to:

At runtime, the conversation will show "The chest contains Axe."
You can set the dialogue database variable in your own C# or UnityScript script with this code:
Code: Select all
using PixelCrushers.DialogueSystem;
...
DialogueLua.SetVariable("chestContents", "Axe");
Option 2:
At runtime, the Dialogue System keeps its data in Lua. In most cases, you don't have to think about this. But sometimes it's helpful to use Lua. For example, you can use the [lua(...)] tag in Dialogue Text:

This is a very powerful feature because you can register your own C# code with Lua. (There is a starter template script in Assets / Dialogue System / Scripts / Templates.)
For example, you can register a function named GetChestContents() that returns the name of the object inside the chest:
Code: Select all
using UnityEngine;
using PixelCrushers.DialogueSystem;
public class MyChest : MonoBehaviour {
public string contents = "Axe"; //<-- Your variable.
void OnEnable() {
Lua.RegisterFunction("GetChestContents", this, SymbolExtensions.GetMethodInfo(() => GetChestContents()));
}
void OnDisable() {
Lua.UnregisterFunction("GetChestContents");
}
public string GetChestContents() {
return contents;
}
}

Re: Scripts variables in Conversations
Hi I just found this solution but I'm curious for option 1, is there a similar option for item and actor? if I have an amount number in an item and I want to print it in the text in dialogue, how can I write it without using the second option(the lua code one)?
thanks!
thanks!
Re: Scripts variables in Conversations
Hi,
Use the [lua(code)] markup tag. For example, say the Axe item has a custom Number field named Cost. You can use this text:
Use the [lua(code)] markup tag. For example, say the Axe item has a custom Number field named Cost. You can use this text:
- Dialogue Text: "The Axe costs [lua(Item["Axe"].Cost)]."