
How to make Achievements?
-
- Posts: 80
- Joined: Sun Jul 17, 2016 3:38 pm
Re: How to make Achievements?
Thanks a lot! 

Re: How to make Achievements?
Hi,
Here's an achievements example:
VN_AchievementsExample_2016-08-19.unitypackage
It imports into Dialogue System Extras/Visual Novel Framework/Example/Achievements Example. You must add two scenes to your build settings: Achievements Example Start and Achievements Example Gameplay. Then play Achievements Example Start.
If you choose the "creep" options ("Enough talk. Let's make out." or "You could always take it off."), you'll get the "Made Girl Angry" achievement:
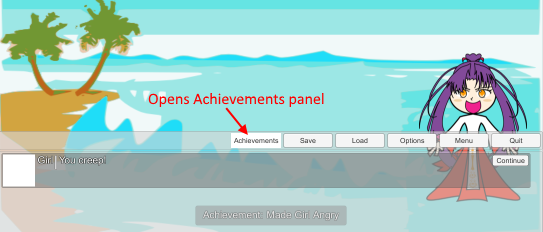
When you click the Achievements button, it opens the Achievements panel:
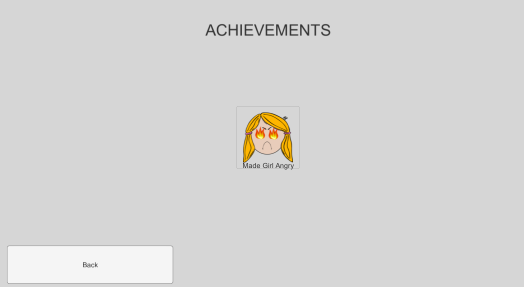
In the dialogue database, I set the "You creep!" entry's Script to:
Here's an achievements example:
VN_AchievementsExample_2016-08-19.unitypackage
It imports into Dialogue System Extras/Visual Novel Framework/Example/Achievements Example. You must add two scenes to your build settings: Achievements Example Start and Achievements Example Gameplay. Then play Achievements Example Start.
If you choose the "creep" options ("Enough talk. Let's make out." or "You could always take it off."), you'll get the "Made Girl Angry" achievement:
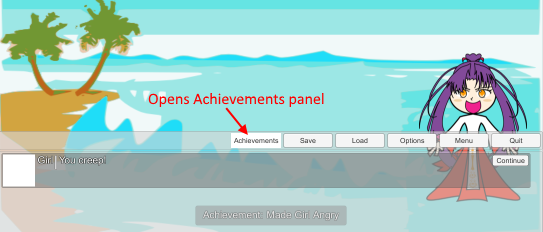
When you click the Achievements button, it opens the Achievements panel:
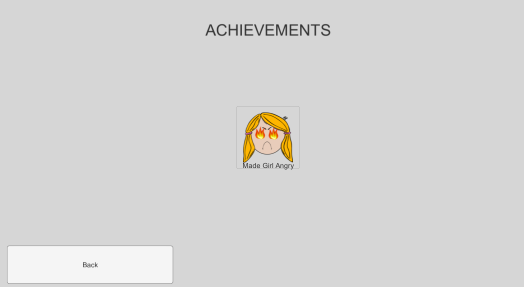
In the dialogue database, I set the "You creep!" entry's Script to:
- Script:
Code: Select all
Variable["Achievement_MadeGirlAngry"] = true; ShowAlert("Achievement: Made Girl Angry")
- Sequence Trigger: Set to OnEnable. Uses SetActive(Achievement_MadeGirlAngry) to show the achievement if this Lua condition is true:
Code: Select all
Variable["Achievement_MadeGirlAngry"]==true
- Sequence Trigger: Set to OnEnable. Uses SetActive(Achievement_MadeGirlAngry,false) to hide the achievement if this Lua condition is true:
Code: Select all
Variable["Achievement_MadeGirlAngry"]==false
-
- Posts: 80
- Joined: Sun Jul 17, 2016 3:38 pm
Re: How to make Achievements?
Yes, I want to know. Please teach me. 

Re: How to make Achievements?
Here's an example that uses PlayerPrefs:
VN_PlayerPrefsAchievementsExample_2016-08-22.unitypackage
The files are in Dialogue System Extras > Visual Novel Framework > Example > PlayerPrefs Achievements Example.
To use it in your own project:
1. Import the Scripts folder from PlayerPrefs Achievements Example.
2. Add an Achievements Panel to the Dialogue Manager's Canvas. Add UI elements for each achievement. Add a "close" button to the Achievements Panel, and set its OnClick() event to: Achievements Panel: GameObject.SetActive: unticked. (See the example PlayerPrefs Achievements Example Start scene.)
3. Add the Achievements script to your Dialogue Manager. Add achievements to the Achievements list. Give each one a name and assign the UI element from the Achievements Panel.
4. Add an Achievements button to your Start Panel > MenuButtons. The OnClick() event should have two entries:
To reset the achievements, inspect the Achievements script on the Dialogue Manager, and click "Reset Achievements".
The Achievements script is below for your reference. There's also a very simple editor script that adds the Reset Achievements button to the Achievements inspector.
VN_PlayerPrefsAchievementsExample_2016-08-22.unitypackage
The files are in Dialogue System Extras > Visual Novel Framework > Example > PlayerPrefs Achievements Example.
To use it in your own project:
1. Import the Scripts folder from PlayerPrefs Achievements Example.
2. Add an Achievements Panel to the Dialogue Manager's Canvas. Add UI elements for each achievement. Add a "close" button to the Achievements Panel, and set its OnClick() event to: Achievements Panel: GameObject.SetActive: unticked. (See the example PlayerPrefs Achievements Example Start scene.)
3. Add the Achievements script to your Dialogue Manager. Add achievements to the Achievements list. Give each one a name and assign the UI element from the Achievements Panel.
4. Add an Achievements button to your Start Panel > MenuButtons. The OnClick() event should have two entries:
- Achievements Panel: GameObject.SetActive: ticked
- Achievements (the script you just added to the Dialogue Manager): UpdateIcons.
- Achievements Panel: GameObject.SetActive: ticked
- Achievements (the script you just added to the Dialogue Manager): UpdateIcons.
Code: Select all
AddAchievement("MadeGirlAngry");
ShowAlert("Achievement: Made Girl Angry")
The Achievements script is below for your reference. There's also a very simple editor script that adds the Reset Achievements button to the Achievements inspector.
Code: Select all
using UnityEngine;
using System;
using System.Collections.Generic;
using PixelCrushers.DialogueSystem;
public class Achievements: MonoBehaviour
{
[Serializable]
public class Achievement
{
public string name;
public GameObject icon;
}
public List<Achievement> achievements = new List<Achievement>();
private void Start()
{
Lua.RegisterFunction("AddAchievement", this, SymbolExtensions.GetMethodInfo(() => AddAchievement(string.Empty)));
UpdateIcons();
}
public void AddAchievement(string achievementName)
{
if (string.IsNullOrEmpty(achievementName))
{
Debug.LogError("AddAchievement was provided a blank achievement name");
}
else if (achievements.Find(x => string.Equals(x.name, achievementName)) == null)
{
Debug.LogError("Achievement '" + achievementName + "' is not defined in the Achievements component");
}
else
{
PlayerPrefs.SetInt(achievementName, 1);
}
}
public void UpdateIcons()
{
foreach (var achievement in achievements)
{
if (achievement != null)
{
if (string.IsNullOrEmpty(achievement.name))
{
Debug.LogError("Achievement name is blank");
}
else if (achievement.icon == null)
{
Debug.LogError("Achievement '" + achievement.icon + "' icon is unassigned");
}
else
{
var hasAchievement = PlayerPrefs.GetInt(achievement.name, 0) == 1;
achievement.icon.SetActive(hasAchievement);
}
}
}
}
}
-
- Posts: 80
- Joined: Sun Jul 17, 2016 3:38 pm
Re: How to make Achievements?
Thanks a lot! 
I'll comeback if I finish it. Thanks again

I'll comeback if I finish it. Thanks again

Re: How to make Achievements?
Hi Tony,
I tried to download the examples, but they are missing prefabs. I guess because they are not compatible with VN framework 2.0. Anyway, I was trying to follow the example, but I don't know how to open the achievement panel, as it looks it's not supported by the menus' script.
I tried to modify the script myself, but I was getting a few errors so I gave up.
Btw, if you have to modify the menu to allow the field of achievements, can I suggest to create also the field to open a log panel? It's quite common in the VN and I saw in the extras there is an example. (although doesn't work either)
Thanks!
I tried to download the examples, but they are missing prefabs. I guess because they are not compatible with VN framework 2.0. Anyway, I was trying to follow the example, but I don't know how to open the achievement panel, as it looks it's not supported by the menus' script.
I tried to modify the script myself, but I was getting a few errors so I gave up.

Btw, if you have to modify the menu to allow the field of achievements, can I suggest to create also the field to open a log panel? It's quite common in the VN and I saw in the extras there is an example. (although doesn't work either)
Thanks!
Re: How to make Achievements?
Hi,
Good ideas. I'll try to update the VN framework soon to:
1. Have an optional achievements panel, and
2. Have a button to open the quest log window.
Good ideas. I'll try to update the VN framework soon to:
1. Have an optional achievements panel, and
2. Have a button to open the quest log window.
Re: How to make Achievements?
Thanks!
Re: How to make Achievements?
Hi,
I was thinking about achievements today, and to improve re-playability it could be a good idea to keep the unlocked achievements in that state in case the player decides to replay the game. Some of those achievements might not be in the "golden path" and still, it would be good to allow him to add them to the list of already unlocked achievements.
Does it make sense? Anyway, just an idea to make the system better.
I was thinking about achievements today, and to improve re-playability it could be a good idea to keep the unlocked achievements in that state in case the player decides to replay the game. Some of those achievements might not be in the "golden path" and still, it would be good to allow him to add them to the list of already unlocked achievements.
Does it make sense? Anyway, just an idea to make the system better.

Re: How to make Achievements?
In that case, achievements should not be part of a saved game. They should be in a separate file or PlayerPrefs. For platform compatibility, I'll go with PlayerPrefs.