Best way to update a slider value from DS
-
- Posts: 32
- Joined: Wed Nov 14, 2018 8:27 pm
Best way to update a slider value from DS
Hi again!
I am trying to figure out the best way to go about doing this - I want to have several sliders (to represent player "stats" in game) and want to change the value of those sliders with variables (ie, when I set the variable PlayerStrength to be +5 it will be represented on the slider)
Should I be writing a custom sequencer command to be updating the slider value through Dialogue system? Or is there a way to do it with Lua script? I admit I am not terribly familiar with either, but I at least want to know which direction I should focus on while trying to work it out.
Thanks!
I am trying to figure out the best way to go about doing this - I want to have several sliders (to represent player "stats" in game) and want to change the value of those sliders with variables (ie, when I set the variable PlayerStrength to be +5 it will be represented on the slider)
Should I be writing a custom sequencer command to be updating the slider value through Dialogue system? Or is there a way to do it with Lua script? I admit I am not terribly familiar with either, but I at least want to know which direction I should focus on while trying to work it out.
Thanks!
Re: Best way to update a slider value from DS
It's probably best to write a C# method and register it with Lua. In this C# method, update the slider value and set the corresponding Dialogue System variable. For example:
If you register this function with Lua, you can call it from a dialogue entry's Script field or from your own C# scripts and/or UnityEvents.
Side note: You can use DialogueLua.SetActorField("Player", "Strength", value) to set a custom field in the Player actor instead of a variable if you prefer.
I'm happy to provide more details if you have questions about this.
Code: Select all
public void SetStrength(int value)
{
strengthSlider.value = value;
DialogueLua.SetVariable("PlayerStrength", value);
}
Side note: You can use DialogueLua.SetActorField("Player", "Strength", value) to set a custom field in the Player actor instead of a variable if you prefer.
I'm happy to provide more details if you have questions about this.
-
- Posts: 32
- Joined: Wed Nov 14, 2018 8:27 pm
Re: Best way to update a slider value from DS
I'm going to read up on how to register with Lua, but in the meantime, I have a couple questions.
Am I attaching this c# script containing the method onto the slider UI element itself? And if so, that would mean I'd attach a similar script to every individual stat slider with their related variables? (ie, setVariable PlayerKnowledge, etc...)
Am I attaching this c# script containing the method onto the slider UI element itself? And if so, that would mean I'd attach a similar script to every individual stat slider with their related variables? (ie, setVariable PlayerKnowledge, etc...)
Re: Best way to update a slider value from DS
Can you please provide some more details? When will these sliders will be visible? Will be player be able to adjust the sliders, or do they only move when the underlying variable value changes? Any other details that might be relevant?
-
- Posts: 32
- Joined: Wed Nov 14, 2018 8:27 pm
Re: Best way to update a slider value from DS
The idea is to have a UI Panel (called Player Stats or something similar) that pulls up 4-5 sliders that show the player's stats throughout the game. they are not interactable, but rather are just a visual representation of the player's stats I am controlling using variables.
The player will be making choices that will affect these stats as the game goes along.
Player gets a number of choices/responses in any given conversation, each response is tied to a variable which will add or subtract based upon the choice they made.
For example, If my stats are Strength, knowledge, and cunning, and the player chooses the response tied to "cunning", I am adjusting the PlayerCunning variable by +5. I then want the associated slider to add +5 so when the player looks at the Player Stats menu, they can see how their choices are affecting their stats.
Does that make sense?
The player will be making choices that will affect these stats as the game goes along.
Player gets a number of choices/responses in any given conversation, each response is tied to a variable which will add or subtract based upon the choice they made.
For example, If my stats are Strength, knowledge, and cunning, and the player chooses the response tied to "cunning", I am adjusting the PlayerCunning variable by +5. I then want the associated slider to add +5 so when the player looks at the Player Stats menu, they can see how their choices are affecting their stats.
Does that make sense?

Re: Best way to update a slider value from DS
Makes perfect sense! Here's an example: SliderExample_2018-11-19.unitypackage
It uses this script:
The script works with custom fields defined in the Player actor:
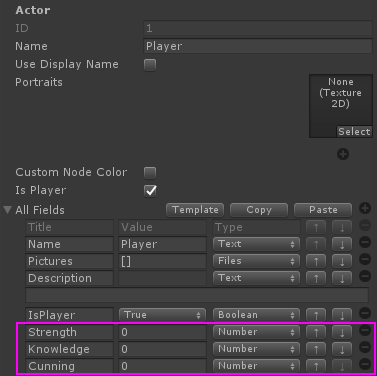
And the dialogue entries' Script fields use the Lua function like this:
It uses this script:
StatsManager.cs
Code: Select all
using UnityEngine;
using PixelCrushers.DialogueSystem;
public class StatsManager : MonoBehaviour
{
public UnityEngine.UI.Slider strengthSlider;
public UnityEngine.UI.Slider knowledgeSlider;
public UnityEngine.UI.Slider cunningSlider;
public string playerActorName = "Player";
private void OnEnable()
{
// Make the functions available to Lua:
Lua.RegisterFunction("ModifyStat", this, SymbolExtensions.GetMethodInfo(() => ModifyStat(string.Empty, (double)0)));
}
private void OnDisable()
{
Lua.UnregisterFunction("ModifyStat");
}
private void Start()
{
SetSliderValues(); // Initialize sliders when starting.
}
private void OnApplyPersistentData()
{
SetSliderValues(); // Also initialize sliders when loading a saved game.
}
public void SetSliderValues()
{
// Initialize slider values from the Dialogue System values:
strengthSlider.value = DialogueLua.GetActorField(playerActorName, "Strength").asInt;
knowledgeSlider.value = DialogueLua.GetActorField(playerActorName, "Knowledge").asInt;
cunningSlider.value = DialogueLua.GetActorField(playerActorName, "Cunning").asInt;
}
public void ModifyStat(string stat, double amount)
{
if (stat == "Strength" || stat == "Knowledge" || stat == "Cunning")
{
var newValue = DialogueLua.GetActorField(playerActorName, stat).asInt + amount;
DialogueLua.SetActorField(playerActorName, stat, newValue);
SetSliderValues();
}
else
{
Debug.LogWarning("Unknown stat: '" + stat + "'.");
}
}
}
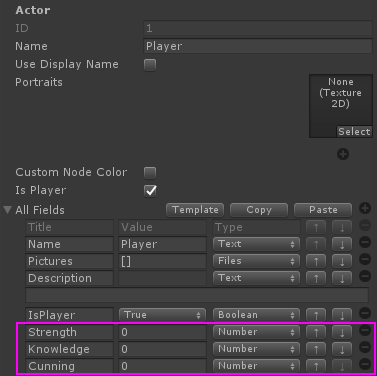
And the dialogue entries' Script fields use the Lua function like this:
Code: Select all
ModifyStat("Strength", 1)
-
- Posts: 32
- Joined: Wed Nov 14, 2018 8:27 pm
Re: Best way to update a slider value from DS
this is absolutely perfect!
Thank you for helping me out! You've definitely got the best support around
Thank you for helping me out! You've definitely got the best support around

Re: Best way to update a slider value from DS
Thanks! Glad to help.
-
- Posts: 32
- Joined: Wed Nov 14, 2018 8:27 pm
Re: Best way to update a slider value from DS
One last question I just thought of - do I need to do anything special to get these values to save with the regular save system?
Re: Best way to update a slider value from DS
Nope! That's the nice thing about saving them in the Dialogue System's data. They're automatically included in saved games.