Hi Tony!
The game I'm working on features animated character closeups: characters can perform animations either in-between lines or randomly while waiting for you to select an option.
To "direct" them I use Articy's "Stage Directions" in DialogueFragments, like the following:
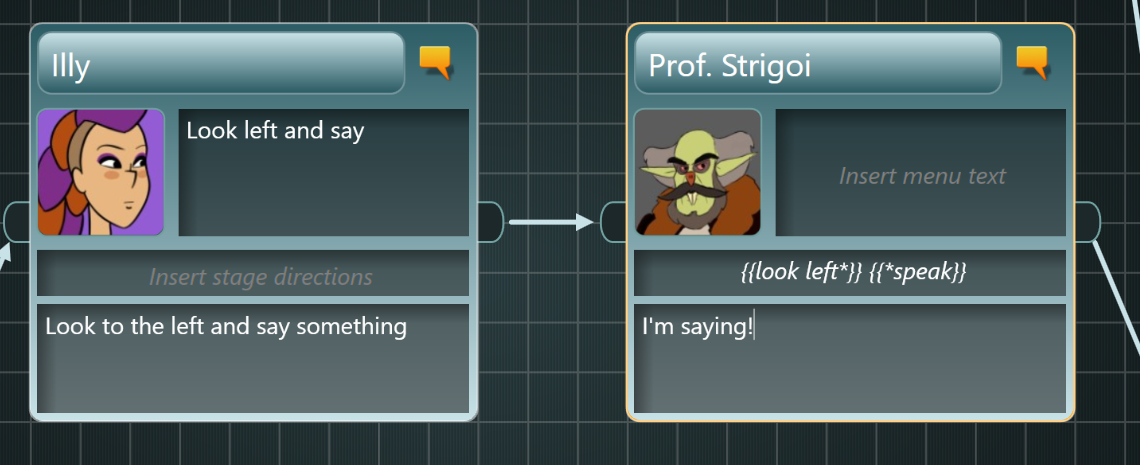
The shortcuts roughly translate to:
Code: Select all
DoTheAnimation()->Message(next); MyCustomAudioWait(entrytag)@Message(next)
But what I see is that while the audio (and related lipsync) do wait for the actual animation to finish, the Subtitle still appears before the animation even starts.
Is there a way to have the subtitle appear along with the audio, without having to create an additional empty line\DialogueFragment? Avoiding it would help a lot when it comes to exporting the voice over spreadsheets and avoid endless "Director_xy_z" empty nodes, I think.
Anyway, I also guess that having it all in a single DialogueFragment would eventually get in the way of me being able to skip the animation only, without skipping the whole dialogue line?
Thanks in advance!